Unit Testing React Components With Jest
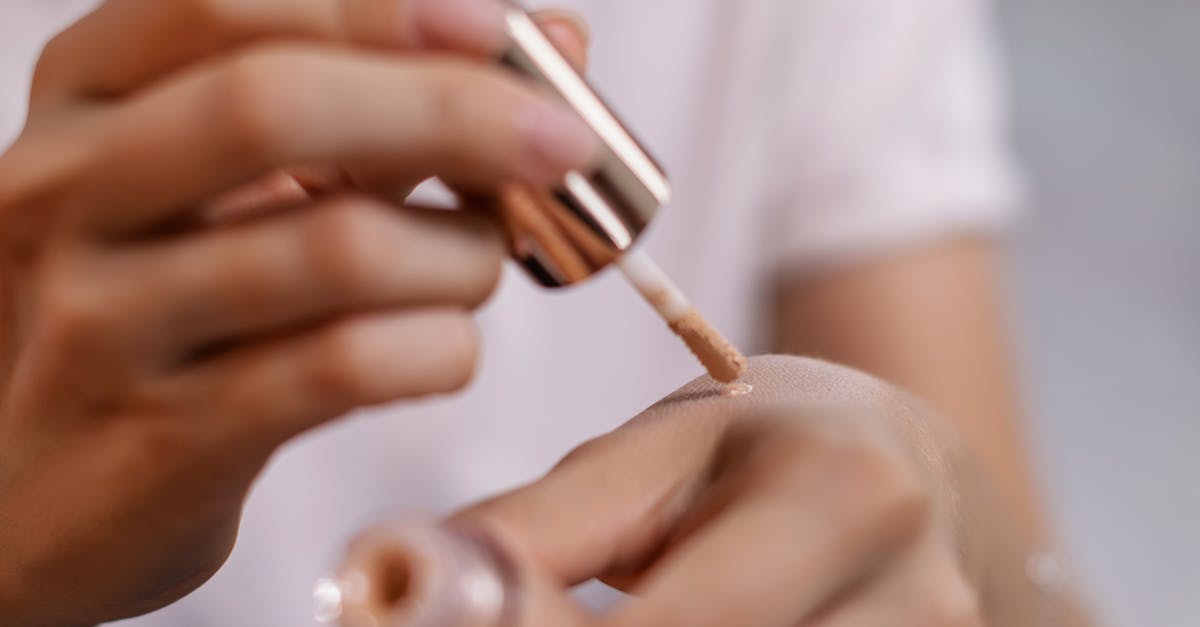
Introduction
Unit testing is an essential part of the software development process. It helps ensure that individual components or units of code perform as expected, even when changes are made. In the context of React, unit testing becomes even more crucial since it allows us to validate the behavior of our components without having to rely solely on manual testing. In this article, we will explore how to effectively unit test React components using Jest.
Why Unit Testing?
Unit testing is like having a safety net for your code. It provides a way to automatically verify that your components are functioning as intended. By writing tests, you can catch bugs and regressions before they make their way into your application's production environment.
Setting Up Jest
Before we jump into writing tests, let's first set up Jest in our React project. Jest is a popular JavaScript testing framework that provides a simple and intuitive API for writing tests. To get started, you'll need to install Jest as a dev dependency:
$ npm install --save-dev jest
Once Jest is installed, you can start writing your tests.
Writing Tests
To effectively test React components with Jest, we need to understand a few key concepts: mocks, assertions, and tests themselves.
Mocks
One of the main advantages of using Jest is its built-in mocking capabilities. Mocking allows us to isolate components and their dependencies, enabling us to test the component in isolation without worrying about external factors. For example, if a component relies on an API call, we can mock the API response and focus solely on testing the component's behavior.
Assertions
Assertions are used to verify the behavior of your code. In the context of Jest, assertions are made using the expect
function. It allows you to check if certain conditions are met and provides several matchers to make your assertions more expressive and concise.
Writing Tests
Now that we have a basic understanding of mocks and assertions, let's dive into writing actual tests. In React, unit tests are typically organized into test files with the .test.js
extension. Let's say we have a Button
component that renders a button element with some text:
import React from 'react';
const Button = ({ text }) => {
return <button>{text}</button>;
};
export default Button;
To test this component, we can create a Button.test.js
file and write our tests:
import React from 'react';
import { render, screen } from '@testing-library/react';
import Button from './Button';
it('renders the button text', () => {
const buttonText = 'Click me';
render(<Button text={buttonText} />);
expect(screen.getByText(buttonText)).toBeInTheDocument();
});
In the example above, we use @testing-library/react
to render our Button
component. We then use the getByText
matcher to assert that the button text is rendered on the screen.
By writing tests like this, we can cover different scenarios and edge cases, ensuring that our components behave as expected in different situations.
Conclusion
Unit testing React components with Jest is a valuable practice that helps us build more robust and reliable applications. By writing tests, you can catch bugs early on, ensure that your components are functioning as intended, and improve the overall quality of your code.